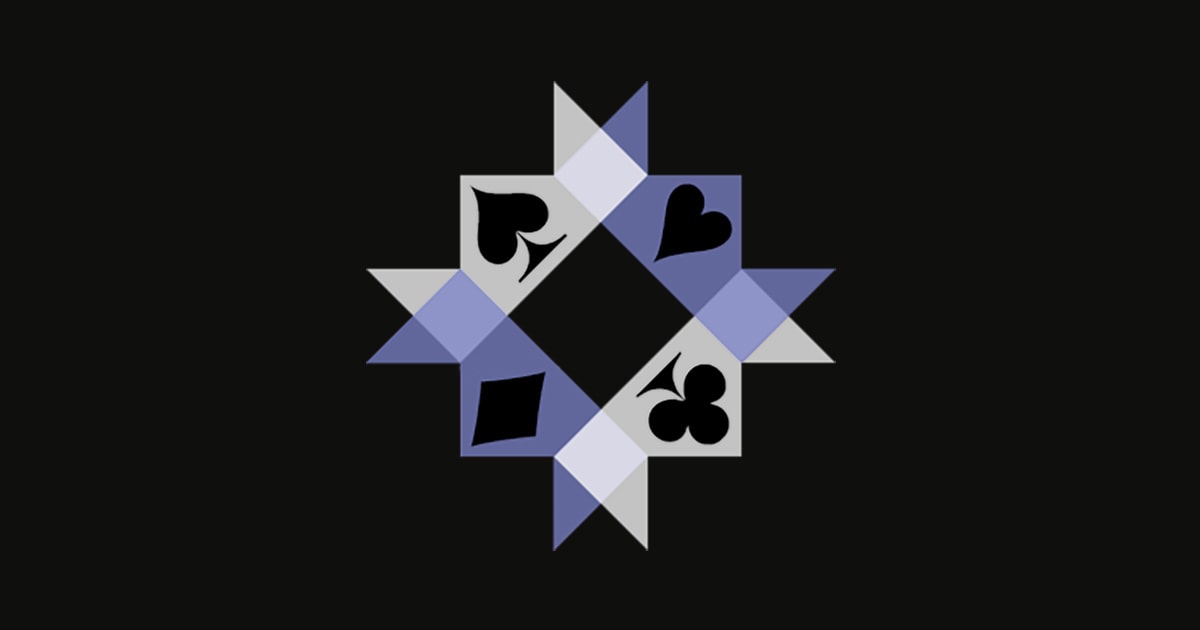
The logo I designed for Kings Corner.
Kings Corner
Learn about Firebase’s onSnapshot listener.
2023-05-26T13:52-07:00
If I were a JavaScript teacher, my final project for the class would ask each student to build a card game of their choice. It would be a great exercise in data structuring – I personally love JavaScript's Set data object for projects like these – and the game logic requires state management and rule checking to ensure each play is valid. I know all this from experience building my own card game.
Last year I taught some friends a game called Kings Corner and discovered it was a hit after each new player couldn't seem to get enough. The easiest way to describe it is "multi-player solitaire" – you can learn more about the rules here after registering for an account.
Thanks to Google's Firebase I was able to quickly set up account authentication and game data storage. What I really needed, however, was a database listener that could push each move update to the user interface in real time. I didn't want players to manually refresh the app to check if it's their turn.
Thankfully, Firebase has onSnapshot. I set up this listener in my React app using the useEffect hook and an empty array of dependencies. This creates the listener when the app initially loads, returning any changes detected in the game's database right after they're committed.
useEffect(
() => {
// load the game and listen for changes
const theDoc = doc(firestore, "Games", gameKey),
unsubscribe = onSnapshot(theDoc, gameplayCallback);
// unsubscribe when the component unmounts
return unsubscribe;
},
[]
);
Now I could see my opponent's moves as they made them, click by click or tap by tap. It was almost as fun as sitting at the table with friends and family – the only thing missing was the lively laughter and conversation, which is why I plan to include a chat feature in the near future.
If no one's online to play, the computer can serve as a worthy adversary. The computer plays using a recursive function that keeps scanning cards until no more valid plays remain. The algorithm was simple to implement yet difficult to beat; I had created a perfectionist player that really keeps you on your toes.
I loved turning a relatively unknown card game into a web app that people anywhere can enjoy, but the best reward has been the joy it's brought my loved ones. Hopefully interest keeps growing. If it does, I would like to start a player mailing list and even look into generating some ad revenue.
My own advertising budget only stretches so far, so if you love Kings Corner would you consider sharing this link with your loved ones? Or if you have your own project ideas and need some help, please send me an email at al@fern.haus.