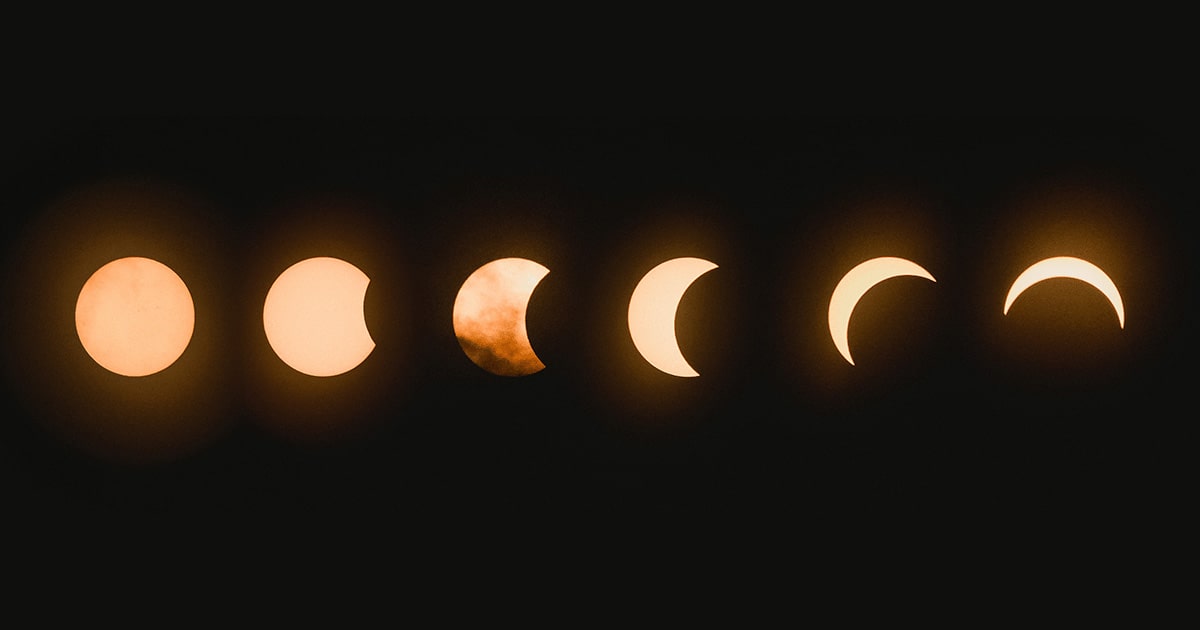
Photo by Mark Tegethoff on Unsplash
Moon-Sun-Tides API
Read the code documentation before you copy and paste.
2023-06-02T13:52-07:00
Creating my own API proved that rarely anything in programming is accomplished alone – instead it's built on the backs of the many engineers that came before. Another lesson it taught me: don't just copy code from Stack Overflow. This online forum is only a starting point, and programmers should always read a solution's original documentation to really understand what they're working with.
The API that I built combined, in my opinion, the most requested time data for natural phenomena: sunsets, sunrises, full and new moons, and tides both high and low. I was surprised a single simple endpoint for all this data didn't already exist. Maybe I didn't dig deep enough, or maybe I didn't want to. What I wanted instead was to create my own.
The online forums helped me find three separate sources for this project:
These endpoints had already done the hard work of observing the natural world. Now all I needed to do was make one simple API from these three more complicated ones.
Stack Overflow had great examples of each one, and all the data seemed correct and uniform with one exception: NOAA's Tides API. The example I found returned dates and times in the coordinates' local timezone (without telling the timezone), but I needed them to be in the same UTC timezone of the sun and moon times.
Side note: If users want to convert UTC timestamps to ones in their local timezones, they can simply create new JavaScript Date objects from the timestamps:
new Date("2023-01-10 13:00 +0");
// prints "Tue Jan 10 2023 05:00:00 GMT-0800 (Pacific Standard Time)"
// since I'm in California.
Solving the problem
This situation got me thinking about how to convert a local time to a UTC timestamp when I knew the coordinates but not the timezone. I came up with the following approach in pseudocode:
- Calculate the timezone offset from UTC (for example, Pacific Standard Time = -8).
- Create a template literal with the local date, time and timezone offset:
`${date} ${time} ${offset}` - Pass the resulting string into a new Date object.
- Get the UTC timestamp from this Date object with the .toUTCString() method.
There is a nifty node package called geo-tz that takes coordinates and returns their timezone offset, which let me successfully implement the pseudocode above. Hooray, the function worked! But did I really need to do all those extra steps?
An easier solution
Even though the approach above works, it's redundant for the tides API. I don't need to convert any timezones because NOAA's API can do it for me with its time_zone parameter. The example endpoint I found on the forums set this value as lst for Local Standard Time, but the original documentation listed another option: gmt for Greenwich Mean Time, aka UTC.I wouldn't have known this was possible if I hadn't studied the code docs. Always review the original documentation for any language, class or API that you use. Stack Overflow is a great tool for pointing programmers in the right direction, but it's up to them to go further. They need to know both that a solution works and how it can work better for their specific project.